“White space is to be regarded as an active element, not a passive background.”
– Jan Tschichold
At least since Apple is using massive white space for their product pages, every web-designer knows that the visitors prefer simple over the complex designs. And I think we can use some of this design principles to make our code more readable.
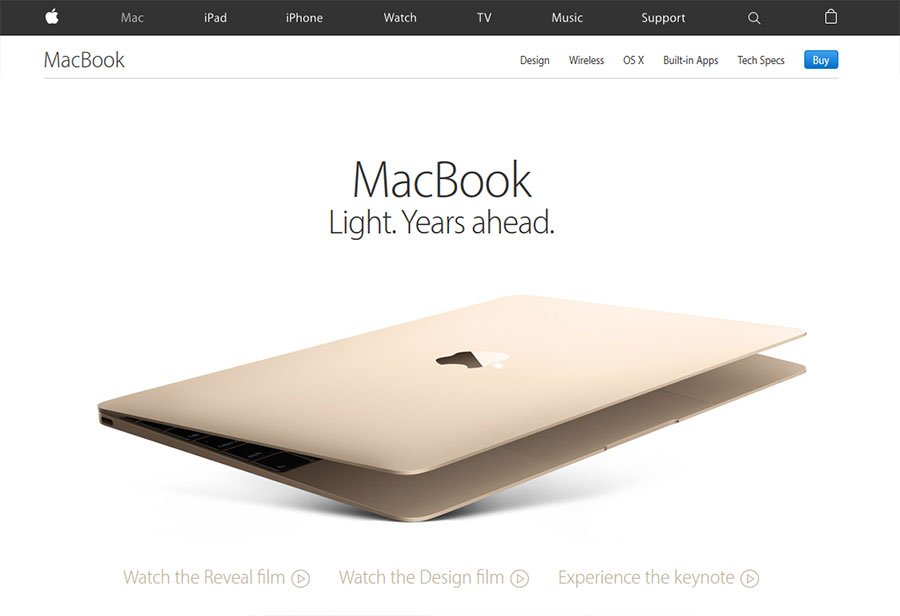
What is white space?
White space is created by pressing the Return key [\n|\r|\r\n], Spacebar key [ ], or the Tab key [\t]. White space is essentially any bit of space in your code that is not taken up by „physical“ characters.
[\n] = LF (Line Feed) → Used as a new line character in Unix/Mac OS X
[\r] = CR (Carriage Return) → Used as a new line character in Mac OS before X
[\r\n] = CR + LF → Used as a new line character in Windows
https://stackoverflow.com/a/15433225/1155858
White space is critical for organizing our code, because we tend to find some relations between “things” to instinctively find a meaning in the composition. Whatever you see, your mind will filter, add and remove the given input and because of that we should prepare the input. So that we can easily consume the code.
- Vertical Whitespace:
- A single blank line.
- Horizontal Whitespace:
- Leading White Space (at the start of a line): Leading white space (i.e., indentation) is addressed elsewhere. (Indent with i.e. 4 spaces is a good default.)
- Internal White Space (in the code itself): The code is more readable with some white space between the different operations in a single line.
- Trailing White Space (at the end of a line): This kind of white spaces are unnecessary and can complicate diffs.
Horizontal alignment: Alignment can aid readability, but it creates problems for future maintenance, if you do not automate your code style.
“White space also makes content more readable. A study (Lin, 2004) found that good use of white space between paragraphs and in the left and right margins increases comprehension by almost 20%. Readers find it easier to focus on and process generously spaced content.” – [Lin, 2004] Evaluating older adults’ retention in hypertext perusal: impacts of presentation media as a function of text topology by Dyi-Yih Michael Lin in “Computers in Human Behavior”, Volume 20, Issue 4, July 2004, Pages 491-503
Where do we use white space?
We mostly add white space after a parameter, function, method, if-, else-, while-, and other calls so that it’s easier to identify where code that belongs together starts and ends. Unlike a book in which you read from top to bottom, we mostly jump into the code and only read parts of the code. It’s more like a newspaper where you read the headline (i.e. method name) and then jump to the next article (i.e. method).
Whitespace changes are tricky for “diff”
You can use something like “git diff -w” …
- –ignore-space-at-eol: Ignore changes in whitespace at EOL.
- -b, –ignore-space-change: Ignore changes in amount of whitespace. This ignores whitespace at line end, and considers all other sequences of one or more whitespace characters to be equivalent.
- -w, –ignore-all-space: Ignore whitespace when comparing lines. This ignores differences even if one line has whitespace where the other line has none.
- –ignore-blank-lines: Ignore changes whose lines are all blank.
… but the problems is still there, that white space changes hurts readability from default diffs in i.e. gitlab / github / etc. so that you should try to commit white space changes in a separated commit.
Be consistent!
The most important and the only things that everybody will agree on is: “Be consistent [and try to automate this process, please]!” For example, don’t mix tabs and spaces or playing different braces for the same type of code. If the team (or project) didn’t use any code style at all, then it’s quite simple, take an existing coding standard (try to follow the conventions already in place for a language, if there are any) where automation is already implemented for and stick to it in the first run.
Design principles for your code
Proximity: Things closer together will be seen as belonging together.
Code: Put code together that belongs together.
bad:
.example_1,.example_2
{
background
:
url
(
images/example.png
)
center center no-repeat
,
url
(
images/example-2.png
)
top left repeat
,
url
(
images/example-3.png
)
top right no-repeat
;}
not that bad:
.example_1,
.example_2 {
background: url(images/example.png) center center no-repeat,
url(images/example-2.png) top left repeat,
url(images/example-3.png) top right no-repeat;
}
I also like to have a single blank line before ‘break’, ‘continue’, ‘declare’, ‘return’, ‘throw’, ‘try’, so that you see that this is some kind of important code. But also the readability of simple “if”-statements can be improved.
bad:
if (($loggedInKunde->bestellungen_genehmigen || $loggedInKunde->manuelle_bestellgenehmigung) && ($wareneingang != 5)) {
not that bad:
if (
(
$loggedInKunde->bestellungen_genehmigen
||
$loggedInKunde->manuelle_bestellgenehmigung
)
&&
$wareneingang != 5
) {
better:
if ($this->useOrderApproval()) {
And please do not mix different things like variable assinment and “if”-statments in one blob of code.
bad:
if (null !== $token = $this->tokenStorage->getToken()) {
not that bad:
$token = $this->tokenStorage->getToken();
if ($token !== null) {
better:
$token = $this->tokenStorage->getToken();
if ($token->exists()) {
Similarity: Things with the same characteristics (shape, color, shading, orientation, …) will be seen as belonging together.
Code: Write code that do similar things in the same code style.
bad:
$valueArray = Bar::searchSplitButKeepQuotes($value);
if (\count($valueArray) <= 0) { return ''; }
$valueFoo = Foo::searchSplit($value);
if (\count($valueFoo) === 0) {
return '';
}
not that bad:
$bars = Bar::searchSplitButKeepQuotes($value);
if (\count($bars) === 0) {
return '';
}
$foos = Foo::searchSplit($value);
if (\count($foos) === 0) {
return '';
}
Symmetry: Our mind tends to perceive objects by finding a starting point and it’s pleased when it can find uniformity structures. Sometimes our mind will see such structures also if they are not really there.
Code: Try to use uniformity code structures.
bad:
if (widget.IsRegular) { service.Process(widget); } else { var result = widget.Calculation(); widget.ApplyResult(result); service.ProcessSpecial(widget); }
not that bad:
if (widget.IsRegular) { service.Process(widget); } else { service.ProcessSpecial(widget); }
Links:
- https://google.github.io/styleguide/
- https://www.smashingmagazine.com/2013/02/using-white-space-for-readability-in-html-and-css/
- https://www.smashingmagazine.com/2009/09/10-useful-usability-findings-and-guidelines/
- https://odetocode.com/blogs/scott/archive/2011/02/07/the-value-of-symmetry.aspx
- https://manifesto.co.uk/design-principles-gestalt-white-space-perception/